This R tutorial describes how to change the point shapes of a graph generated using R software and ggplot2 package.
Related Book:
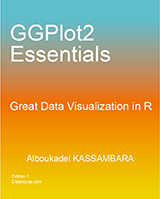
GGPlot2 Essentials for Great Data Visualization in R
Point shapes in R
The different points shapes commonly used in R are illustrated in the figure below :
Create some data
mtcars data is used in the following examples.
df <- mtcars[, c("mpg", "cyl", "wt")]
df$cyl <- as.factor(df$cyl)
head(df)
## mpg cyl wt
## Mazda RX4 21.0 6 2.620
## Mazda RX4 Wag 21.0 6 2.875
## Datsun 710 22.8 4 2.320
## Hornet 4 Drive 21.4 6 3.215
## Hornet Sportabout 18.7 8 3.440
## Valiant 18.1 6 3.460
Make sure to convert the column cyl from a numeric to a factor variable.
Basic scatter plots
Create a scatter plot and change point shapes using the argument shape :
library(ggplot2)
# Basic scatter plot
ggplot(df, aes(x=wt, y=mpg)) +
geom_point()
# Change the point shape
ggplot(df, aes(x=wt, y=mpg)) +
geom_point(shape=18)
# change shape, color, fill, size
ggplot(df, aes(x=wt, y=mpg)) +
geom_point(shape=23, fill="blue", color="darkred", size=3)
Note that, the argument fill can be used only for the point shapes 21 to 25
Scatter plots with multiple groups
Change the point shapes, colors and sizes automatically
In the R code below, point shapes, colors and sizes are controlled automatically by the variable cyl :
library(ggplot2)
# Scatter plot with multiple groups
# shape depends on cyl
ggplot(df, aes(x=wt, y=mpg, group=cyl)) +
geom_point(aes(shape=cyl))
# Change point shapes and colors
ggplot(df, aes(x=wt, y=mpg, group=cyl)) +
geom_point(aes(shape=cyl, color=cyl))
# change point shapes, colors and sizes
ggplot(df, aes(x=wt, y=mpg, group=cyl)) +
geom_point(aes(shape=cyl, color=cyl, size=cyl))
Change point shapes, colors and sizes manually :
The functions below can be used :
- scale_shape_manual() : to change point shapes
- scale_color_manual() : to change point colors
- scale_size_manual() : to change the size of points
# Change colors and shapes manually
ggplot(df, aes(x=wt, y=mpg, group=cyl)) +
geom_point(aes(shape=cyl, color=cyl), size=2)+
scale_shape_manual(values=c(3, 16, 17))+
scale_color_manual(values=c('#999999','#E69F00', '#56B4E9'))+
theme(legend.position="top")
# Change the point size manually
ggplot(df, aes(x=wt, y=mpg, group=cyl)) +
geom_point(aes(shape=cyl, color=cyl, size=cyl))+
scale_shape_manual(values=c(3, 16, 17))+
scale_color_manual(values=c('#999999','#E69F00', '#56B4E9'))+
scale_size_manual(values=c(2,3,4))+
theme(legend.position="top")
Infos
This analysis has been performed using R software (ver. 3.1.2) and ggplot2 (ver. 1.0.0)